Understanding React Native for Mobile Development
What Is React Native?
React Native is a popular framework for building mobile apps using JavaScript and React.
Developed by Facebook, it allows for the creation of natively rendered mobile applications for iOS and Android with a single codebase.
React Native uses the same design principles as React but applies them to mobile UI components, enabling a seamless development experience across platforms.
With its robust set of features, developers can write once and deploy their applications to multiple platforms.
- Cross-Platform Compatibility: Write one codebase for both iOS and Android, reducing development time and costs.
- Hot Reloading: View changes instantly while maintaining the app’s state, speeding up the development process.
- Rich Ecosystem: Access a wide range of libraries and third-party plugins to extend app functionality.
- Strong Community Support: Benefit from active community contributions and extensive documentation.
- Native Performance: Achieve near-native performance by using native components for rendering.
Key Features of React Native
Live Reload and Hot Reloading
React Native includes Live Reload and Hot Reloading, which streamline the development process. Live Reload automatically reloads the app when changes are saved.
If I update a file, Live Reload can reflect these changes instantly. Hot Reloading, on the other hand, keeps the app running while injecting new versions of the files that I modified.
This speeds up the development cycle by maintaining the app’s state and providing immediate feedback.
Access to Native APIs
React Native offers direct access to native APIs, which allows developers to integrate platform-specific features into their apps.
Through bridges, I can use native modules in Java or Swift within a React Native app.
This means I can leverage features like camera access, geolocation, and push notifications, ensuring a richer user experience.
Integrating these native components aids in achieving near-native performance and extensive functionality, which is essential for modern mobile applications.
Setting Up Your Development Environment
Installing Necessary Tools and SDKs
Getting your development environment ready involves installing several tools and SDKs. First, ensure that Node.js is installed on your machine.
Node.js provides the runtime environment for running your application, and npm (Node Package Manager) comes bundled with it. Visit nodejs.org to download and install it.
Next, install React Native CLI. Open a terminal window and run the following command:
npm install -g react-native-cli
To build Android apps, download and install Android Studio.
This IDE includes Android SDKs, which are essential for Android app development. During the installation, ensure to check the boxes for “Android SDK,” “Android SDK Platform,” and “Android Virtual Device.”
For iOS app development, ensure Xcode is installed from the Mac App Store.
Xcode provides the necessary tools to build, test, and run iOS apps. After installation, agree to the licensing terms and set up the command-line tools by running:
sudo xcode-select --switch /Applications/Xcode.app/Contents/Developer
sudo xcodebuild -runFirstLaunch
Configuring an IDE for React Native Development
An Integrated Development Environment (IDE) streamlines the development process. Popular choices include Visual Studio Code, Atom, and WebStorm.
Visit the IDE’s official website and download the installer.
Here’s a quick overview of setting up Visual Studio Code:
- Download Visual Studio Code from code.visualstudio.com.
- Install the React Native Tools extension for debugging and IntelliSense support.
- Install the ESLint extension to enforce coding standards.
For Android development with Android Studio:
- Open Android Studio.
- Navigate to
File > Settings > Appearance & Behavior > System Settings > Android SDK
. - Ensure the required SDKs and tools are available.
For iOS development with Xcode:
- Open Xcode.
- Go to
Preferences > Locations
. - Select the current version under the Command Line Tools dropdown.
This setup process ensures you have a robust environment tailored for React Native development, enabling efficient cross-platform mobile application creation.
Building Your First App
Creating a New Project
To create a new project, use the React Native CLI. Open your terminal and type npx react-native init MyFirstApp
.
This command generates a new React Native project named “MyFirstApp” with a standard structure including folders for Android and iOS code. Inside the App.js
file, you’ll find a basic template to get started quickly.
Understanding Basic Components
React Native offers core components such as View
, Text
, Image
, and Button
. Use View
to create containers, Text
to display text, Image
to show images, and Button
to create interactive elements.
For instance, include a simple text component like this:
import React from 'react';
import { View, Text } from 'react-native';
const App = () => {
return (
<View>
<Text>Hello, World!</Text>
</View>
);
};
export default App;
Adding Navigation
For navigation, use a popular library like React Navigation. Install it with npm install @react-navigation/native
. Then, install necessary dependencies:
npm install react-native-screens react-native-safe-area-context
npm install @react-navigation/stack
Configure your app to use a stack navigator:
import 'react-native-gesture-handler';
import * as React from 'react';
import { NavigationContainer } from '@react-navigation/native';
import { createStackNavigator } from '@react-navigation/stack';
import HomeScreen from './screens/HomeScreen';
import DetailsScreen from './screens/DetailsScreen';
const Stack = createStackNavigator();
const App = () => {
return (
<NavigationContainer>
<Stack.Navigator initialRouteName="Home">
<Stack.Screen name="Home" component={HomeScreen} />
<Stack.Screen name="Details" component={DetailsScreen} />
</Stack.Navigator>
</NavigationContainer>
);
};
export default App;
This code sets up navigation between “HomeScreen” and “DetailsScreen”.
Testing and Debugging Your App
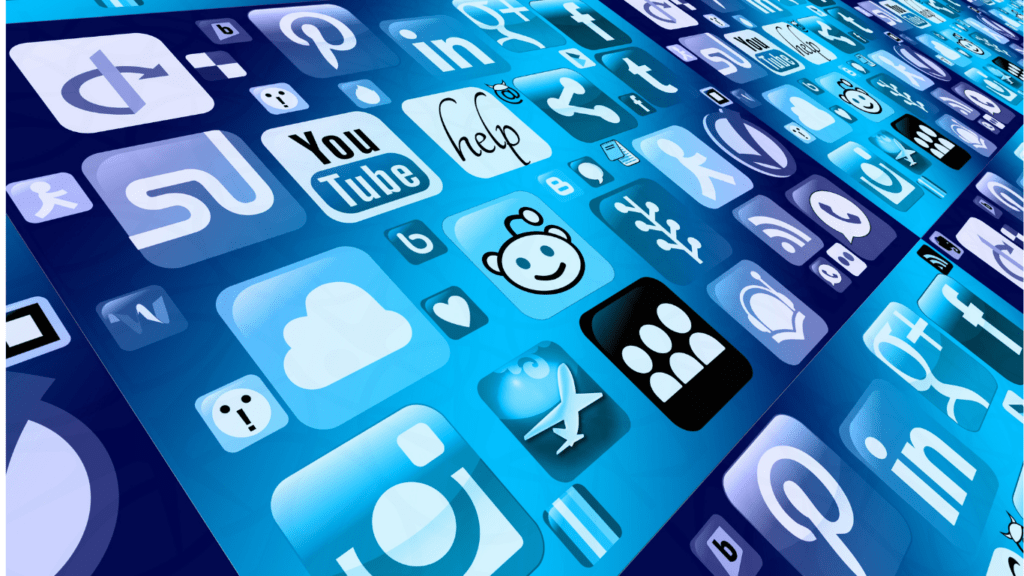
Using the Simulator for iOS and Android
Simulating your app on both iOS and Android environments ensures that it works as expected on different devices. For iOS, you can use Xcode’s built-in simulator.
After starting the simulator in Xcode, run react-native run-ios
in your project directory to launch the app.
This command builds and runs the app on the simulator, reflecting changes in real time if you save updates in your code.
For Android, Android Studio offers robust simulation features. Start an Android Virtual Device (AVD) via the AVD Manager.
Then, use react-native run-android
in your terminal to deploy the app.
The AVD will display your app, allowing you to test its functionality. Be mindful of device-specific behaviors and ensure your app’s UI and features perform consistently across both platforms.
Debugging with React Native Tools
Debugging is essential to identify and fix issues in your app. React Native provides several built-in tools for debugging.
Using the Chrome Developer Tools, you can inspect elements, set breakpoints, and monitor network requests.
Enable this by shaking your device (or Ctrl+M on an Android emulator, Cmd+D on an iOS emulator) and selecting “Debug JS Remotely.”
React Native Debugger offers an enhanced debugging experience.
It includes Redux DevTools for state management, making it easier to track state changes. Install React Native Debugger and start it alongside your app for a comprehensive debug environment.
Reactotron is another powerful tool. It assists with logging, state management, and tracking API requests. Integrate Reactotron into your project to streamline troubleshooting.
By using these tools effectively, you can diagnose and resolve issues promptly, ensuring a smooth user experience.
Deploying Your App to App Stores
Preparing Your App for Submission
Preparing an app for submission involves several steps to ensure it meets the necessary requirements. Create a release build for both iOS and Android platforms. For iOS, use Xcode to archive your app and export it as an .ipa file.
For Android, generate a signed APK using Android Studio. Ensure that your app’s metadata, including the app’s title, description, and keywords, are optimized for discoverability.
Review your app’s design and functionality to ensure it aligns with the Human Interface Guidelines (HIG) for iOS and the Material Design Guidelines for Android.
Test the app on actual devices to check for any performance issues or bugs that might not appear in simulators.
Verify that your app’s permissions and privacy policies are transparent, and provide detailed information on how user data is handled. Many app stores require this information to protect user privacy.
Navigating the App Store and Google Play Guidelines
Submitting an app to app stores requires compliance with specific guidelines.
For Apple’s App Store, adhere to the App Store Review Guidelines.
Ensure your app doesn’t have prohibited content and follows guidelines for safety, performance, business, design, and legal aspects. Utilize TestFlight for beta testing.
For Google Play, follow the Google Play Developer Program Policies.
Focus on the app content, functionality, and user experience, ensuring your app adheres to appropriate content ratings and includes required consent dialogs.
Use the Google Play Console for alpha and beta testing.
Keep track of submission deadlines and possible review times, which may vary. An app review process might take several days, so plan your release appropriately.
By covering these aspects, you’ll be well-prepared to deploy your React Native app to both the App Store and Google Play.