Understanding Functional Programming
What Is Functional Programming?
Functional programming (FP) is a programming paradigm that treats computation as the evaluation of mathematical functions. FP avoids changing state and mutable data, focusing instead on immutability and pure functions.
In FP, functions are first-class citizens, meaning you can pass them as arguments, return them from other functions, and assign them to variables.
- Immutability: In FP, data structures are immutable. Once created, their state cannot change. This leads to more predictable and bug-free code.
- Pure Functions: Pure functions always produce the same output for the same input and have no side effects. For example, a function adding two numbers returns their sum without modifying any external state.
- First-Class and Higher-Order Functions: Functions in FP can be assigned to variables and passed as arguments. Higher-order functions take other functions as arguments or return them as results.
- Function Composition: FP emphasizes combining simple functions to build more complex ones. This modularity enhances code reusability and readability.
- Lazy Evaluation: Only evaluate expressions when their results are needed, which conserves resources and improves performance.
Understanding these principles is crucial to mastering functional programming and recognizing its benefits in modern software development.
Key Benefits of Functional Programming
Improved Code Clarity
Functional programming promotes clear and understandable code. By using pure functions, which do not rely on external state or cause side effects, I can ensure that each function’s behavior is consistent and predictable.
This makes the codebase easier to read and maintain, as each function operates independently of others.
For example, given a function that adds two numbers, the output will always be the same for the same inputs, without modifying any external variables.
Easier Testing and Debugging
With functional programming, testing and debugging become straightforward. Since pure functions do not have side effects, I can write unit tests that accurately verify each function’s behavior.
This predictability reduces the likelihood of bugs caused by changing state. For instance, when testing a function that transforms a list of numbers, I only need to check the return value against expected results without worrying about hidden dependencies.
Enhancing Code Reusability
Functional programming enhances reusability through higher-order functions and function composition.
Higher-order functions take other functions as arguments or return them, enabling me to create modular and reusable code components.
Function composition allows me to build complex operations by combining simpler functions.
For example:
- chaining functions that filter
- map
- reduce a list can transform data efficiently and flexibly
- promoting code reuse and reducing redundancy
Popular Functional Programming Languages
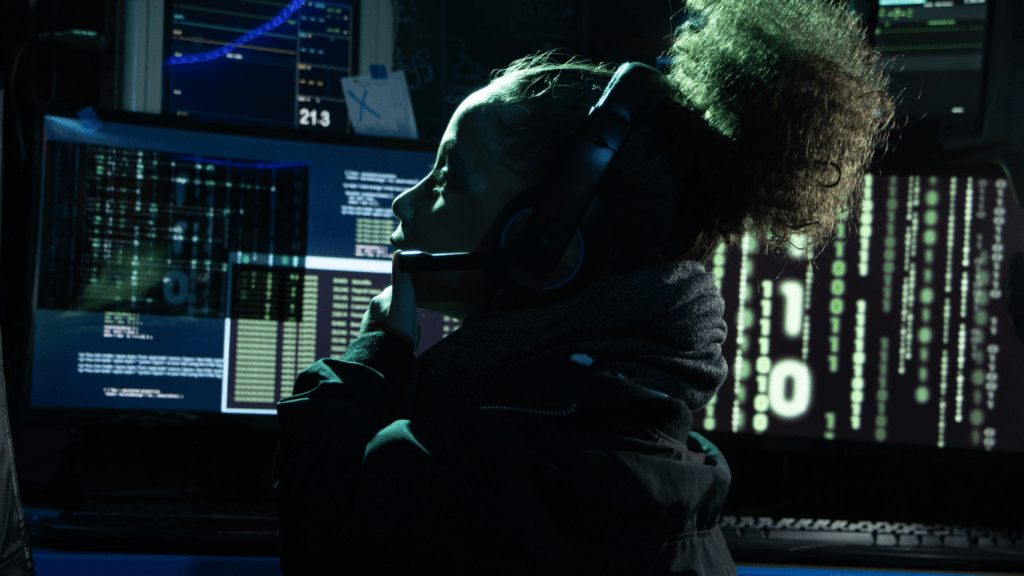
Clojure
Clojure is a modern, dynamic language that builds on the Lisp programming language. It runs on the Java Virtual Machine (JVM) and integrates seamlessly with Java.
Clojure emphasizes immutability and persistent data structures, making it well-suited for building concurrent systems. Its syntax is simple and homoiconic, allowing developers to treat code as data.
I find Clojure particularly effective for real-time systems due to its robust concurrency features.
Haskell
Haskell is a statically typed, purely functional programming language known for lazy evaluation.
It uses a sophisticated type system with type inference, allowing for code safety and correctness. Haskell’s syntax is terse and expressive, enabling developers to write concise programs.
One of its strengths is in domains like data analysis and financial modeling where mathematical correctness is crucial. In my experience, Haskell’s strong type system helps catch errors at compile-time, reducing runtime exceptions.
Scala
Scala is a hybrid functional and object-oriented language designed to work on the JVM.
It integrates with Java libraries, tools, and frameworks, making it a versatile choice for functional programming within enterprise environments.
Scala’s syntax supports concise and readable code, blending functional and imperative styles seamlessly.
Its powerful type inference, along with features like pattern matching and higher-order functions, increases developer productivity.
I utilize Scala often when transitioning teams from Java to functional programming due to its interoperability and ease of adoption.
Functional Programming in Industry
Case Studies: Real-World Applications
Functional programming (FP) has seen increasing adoption in various industries due to its benefits like immutability and pure functions.
For example, Twitter uses Scala for its scalability and functional features to handle vast amounts of data.
Walmart leverages Haskell for its performance and reliability in handling high-concurrency environments during peak shopping seasons.
Spotify employs Clojure for data processing pipelines, benefiting from its immutable data structures and concurrency support.
Industry Adoption Trends
FP’s presence in industry varies across sectors. Tech giants like Google, Amazon, and Microsoft have incorporated FP principles into their systems to enhance code maintainability and reliability.
Financial institutions adopt FP to reduce bugs in mission-critical software. According to the 2022 Stack Overflow Developer Survey, 37% of developers reported using FP features regularly, reflecting its growing importance.
Open-source communities and startups are also increasingly using FP languages, fostering innovation and more maintainable code practices.