Understanding the Basics of JavaScript
Key Concepts Every Developer Should Know
JavaScript operates as a high-level, interpreted programming language. It’s integral for enhancing user interactions on websites. Core concepts include variables, functions, and prototypes.
Variables store data values and exist in three forms: var
, let
, and const
. While var
has function scope, let
and const
have block scope.
Functions, both named and anonymous, encapsulate code and enable reusability.
Prototypes act as blueprints for creating objects, supporting JavaScript’s object-oriented capabilities.
Event handling is another crucial area.
JavaScript uses events to respond to user actions, such as clicks or keyboard input. DOM manipulation allows developers to dynamically alter HTML and CSS, creating interactive web pages.
It’s essential to grasp these basics to build functional, engaging applications efficiently.
Common Misconceptions and Errors in JavaScript
JavaScript’s loosely-typed nature leads to misunderstandings. One common misconception is its equality operators.
The ==
operator performs type coercion, which can produce unexpected results, unlike ===
that checks both value and type.
For instance, 3 == '3'
is true, but 3 === '3'
is false.
Another area is scoping. Using var
for variable declaration can lead to scope-related bugs, as it has function scope, potentially overriding variables unintentionally. let
and const
mitigate this issue by providing block scope.
An off-quoted error is the use of global variables, which can cause conflicts and unpredictable behavior in code. Avoiding global variables enhances code modularity and reduces conflicts.
Async operations often confuse developers. JavaScript’s single-threaded nature means asynchronous processes must be properly managed using Promises or async/await syntax.
Ignoring this can result in callback hell or unresponsive interfaces. Understanding these errors and misconceptions is critical for mastering JavaScript and advancing front-end development skills.
Mastering JavaScript: Essential Tips for Developers
Writing Clean and Efficient Code
Writing clean and efficient code is crucial in JavaScript development. I always recommend using ES6+ features like let
and const
for variable declarations.
They help avoid issues related to variable hoisting and scope. Adopting arrow functions enhances the readability and conciseness of the code.
Code modularity is another practice I follow. I use modules and imports to divide code into reusable pieces, which simplifies maintenance.
Implementing functional programming principles can reduce side effects and make the code more predictable.
Pure functions, for example, don’t depend on or modify the state, which leads to fewer bugs and easier debugging.
I also ensure to follow consistent naming conventions and use meaningful variable and function names. This improves code readability and makes it easier for others to understand the codebase.
Adopting a consistent code style and using linters like ESLint helps enforce these standards.
Debugging and Error Handling Techniques
Effective debugging and error handling are essential for robust JavaScript applications. I use the browser’s built-in developer tools extensively. The console helps to log errors, warnings, and informational messages.
Setting breakpoints allows me to pause execution and inspect variables at specific points, aiding in identifying issues.
In addition to basic debugging, I implement comprehensive error handling. Using try...catch
blocks helps manage runtime errors gracefully.
For asynchronous code, I prefer using async/await
along with try...catch
to simplify error handling.
It’s important to provide meaningful error messages and log errors for future reference.
To avoid common pitfalls, I use strict mode by including 'use strict';
at the beginning of my scripts. This helps catch common JavaScript errors and enforces a cleaner code syntax.
Additionally, adopting unit tests with frameworks like Jest ensures that individual components work as expected, leading to more reliable and maintainable code.
Tools and Frameworks for Enhancing JavaScript Development
Libraries That Boost Productivity
JavaScript libraries boost productivity by providing pre-written code for common tasks. Three essential libraries stand out:
- Lodash: This utility library simplifies complex JavaScript operations. Lodash offers functions for array manipulation, object management, and string handling. For instance,
_.cloneDeep
creates deep copies of objects, preventing unintended mutations. - Moment.js: Handling dates and times can be cumbersome. Moment.js helps format, validate, and manipulate dates. For example,
moment().format('YYYY-MM-DD')
quickly converts a date object to a standard format. - Axios: Making HTTP requests is straightforward with Axios. It’s a promise-based HTTP client for the browser and Node.js. Using
axios.get('/api/data')
simplifies fetching data from APIs.
Using these libraries streamlines development, allowing me to focus on building features rather than reinventing the wheel.
Choosing the Right Framework: React vs Angular vs Vue
Choosing between React, Angular, and Vue can be challenging. Each framework has unique strengths and use cases:
- React: Developed by Facebook, React focuses on building UIs with reusable components. Its virtual DOM boosts performance.
React is versatile and commonly used in single-page applications (SPAs). JSX syntax combines JavaScript and HTML, enabling component-based architecture. - Angular: Google-backed Angular offers a full-fledged framework with extensive tools for building SPAs.
It uses TypeScript, enhancing code quality with static typing. Angular’s two-way data binding simplifies synchronization between the model and the view.
The Angular CLI speeds up development by scaffolding applications quickly. - Vue: Vue is known for its simplicity and flexibility. Its learning curve is gentle, making it ideal for beginners. Vue supports both declarative rendering and component-based architecture. Vue’s ecosystem, including Vue Router and Vuex, provides tools for routing and state management.
When choosing a framework, consider project requirements, team expertise, and long-term maintenance. Each of these frameworks offers robust solutions for building scalable, high-performance applications.
Advanced Techniques in JavaScript
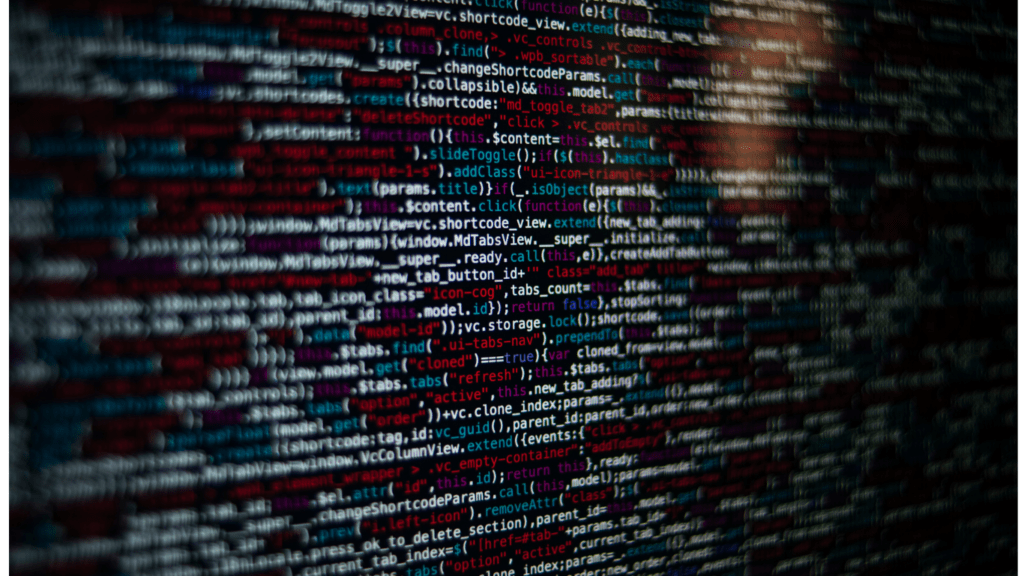
Asynchronous Programming and Promises
Asynchronous programming enables efficient handling of tasks like network requests without blocking the main thread.
Promises simplify managing asynchronous code by representing operations that haven’t yet completed.
Promises have three states: pending, fulfilled, and rejected. To create a promise:
let myPromise = new Promise((resolve, reject) => {
setTimeout(() => {
resolve('Success!');
}, 1000);
});
Using .then()
handles successful resolution, and .catch()
deals with rejections:
myPromise
.then(result => console.log(result))
.catch(error => console.error(error));
For simultaneous execution, combine promises using Promise.all
:
let promise1 = Promise.resolve(3);
let promise2 = 42;
let promise3 = new Promise((resolve, reject) => {
setTimeout(resolve, 100, 'foo');
});
Promise.all([promise1, promise2, promise3]).then(values => {
console.log(values); // Output: [3, 42, 'foo']
});
Leveraging ES6 Features for Better Code
JavaScript ES6 introduced features that enhance code readability and functionality.
Arrow Functions
Arrow functions simplify syntax:
let add = (a, b) => a + b;
Template Literals
Template literals allow embedding expressions:
let name = 'John';
let greeting = `Hello, ${name}!`;
Destructuring Assignment
Destructuring extracts values from arrays or properties from objects:
let [a, b] = [1, 2];
let {name, age} = {name: 'John', age: 30};
Modules
ES6 modules facilitate modularity:
// In myModule.js
export let myVariable = 'value';
export function myFunction() { /* ... */ }
// In another file
import { myVariable, myFunction } from './myModule';
Utilizing these ES6 features, I consistently enhance maintainability and reduce complexity in my front-end development projects.
Staying Updated with JavaScript Trends
Continuous Learning and Community Engagement
Engaging with the JavaScript community keeps me updated on the latest trends. Participating in forums like Stack Overflow and attending meetups provides valuable insights.
Reading blogs from JavaScript experts such as David Walsh, Addy Osmani, and Kyle Simpson offers diverse perspectives and tips.
Subscribing to newsletters like JavaScript Weekly delivers updates on new libraries, frameworks, and tools directly to my inbox.
Future Prospects in JavaScript Development
Understanding future trends in JavaScript helps me stay ahead. The rise of WebAssembly indicates that performance-intensive applications will be more efficient.
Continued evolution of JavaScript frameworks like React, Vue, and Angular enhances front-end capabilities. Watching for updates in ECMAScript standards ensures my code remains modern and efficient.