Understanding the Role of Data Structures in Competitive Programming
Choosing the Right Data Structure for the Problem
Selecting the right data structure is vital to solving programming challenges. Each problem has specific requirements, and certain data structures suit certain tasks better.
For instance, arrays and linked lists handle sequential data effectively, while hash tables provide efficient access to elements using keys.
When facing problems involving hierarchical data, trees (like binary search trees or AVL trees) offer efficient searching and sorting.
For problems requiring fast access and update times, consider balanced trees or heaps.
Recognizing the problem type helps narrow down the appropriate data structure, which can significantly reduce the time complexity and improve execution speed.
Complexity and Performance Analysis
Efficiency in competitive programming hinges on understanding time and space complexity. Evaluating the complexity of chosen data structures helps predict their performance.
- Arrays offer O(1) access time but resizing them is O(n). Linked lists provide O(1) insertion and deletion but have O(n) access time.
- Hash tables typically allow O(1) average-time complexity for operations, but they may degrade to O(n) in worst cases due to collisions.
- Balanced trees (like AVL trees) ensure O(log n) time for searches, insertions, and deletions.
When comparing algorithms, considering this complexity is essential, as it determines how they will scale with larger input sizes.
Key Data Structures You Must Know
Arrays and Strings
Arrays and strings form the backbone of many problems in competitive programming.
Arrays are contiguous memory blocks storing elements of the same data type, while strings are arrays of characters.
They offer efficient indexing and iteration, making them ideal for searching, sorting, and manipulating sequences.
Use arrays for problems requiring random access, and strings for text manipulation challenges (e.g., pattern matching).
Stacks and Queues
Stacks and queues are linear data structures that differ in how elements are added and removed.
A stack follows Last In, First Out (LIFO) order, suitable for problems involving recursion, backtracking, and memory management.
In contrast, queues follow First In, First Out (FIFO) order, often used in scheduling, breadth-first search (BFS), and buffer handling. Each provides distinct methods for managing sequences of elements.
Trees and Graphs
Trees and graphs represent hierarchical and connected structures, respectively.
- Trees, including binary trees and binary search trees (BST), are optimal for hierarchical data representation and efficient searching and sorting.
- Graphs model pairwise relationships between objects and can be directed or undirected, crucial for problems involving navigation, network analysis, and connectivity.
- Mastering traversal algorithms like depth-first search (DFS) and BFS is essential for handling tree and graph-related challenges.
Mastering Essential Algorithms
Sorting and Searching Techniques
Understanding sorting and searching techniques is crucial for competitive programming. Sorting algorithms, such as Quicksort, Mergesort, and Heapsort, are fundamental.
Quicksort, with its average-case time complexity of O(n log n), is often used due to its performance in practice. Mergesort guarantees a stable sort with the same O(n log n) complexity, making it suitable for larger datasets.
Heapsort offers in-place sorting with optimal O(n log n) worst-case time complexity.
Searching algorithms are equally vital. Binary Search, which operates in O(log n) time, is efficient for finding elements in a sorted array.
Linear Search, despite its O(n) time complexity, is useful for smaller or unsorted datasets.
Combining sorting with searching techniques enhances problem-solving efficacy.
Graph Algorithms
Graph algorithms are indispensable for handling complex problems involving nodes and edges.
Depth-First Search (DFS) and Breadth-First Search (BFS) are foundational traversal algorithms. DFS, with O(V+E) complexity, is ideal for exploring all possible paths in a graph, while BFS, also O(V+E), is perfect for finding the shortest path in unweighted graphs.
Shortest path algorithms like Dijkstra’s and Bellman-Ford are necessary for weighted graphs.
Dijkstra’s algorithm, with O(V^2) complexity when using an adjacency matrix, is efficient for non-negative weights.
Bellman-Ford, despite its higher O(VE) complexity, handles graphs with negative weights, making it versatile.
Dynamic Programming
Dynamic programming (DP) addresses problems by breaking them into subproblems and storing results to avoid redundant computations. The two main approaches are memoization (top-down) and tabulation (bottom-up). Memoization uses recursion and caching, while tabulation iteratively builds up solutions.
Classic examples include the Fibonacci sequence, solved in O(n) time using DP, and the Knapsack problem, which employs both 0/1 and fractional approaches.
The Longest Common Subsequence problem, with a O(n*m) DP solution, is indispensable for sequence analysis. Mastery of DP techniques allows for efficient handling of complex problems within competitive programming constraints.
By integrating these essential algorithms—sorting, searching, graph algorithms, and dynamic programming—competitive programmers enhance their problem-solving capabilities, optimizing both time complexity and solution elegance.
Advanced Topics in Competitive Programming
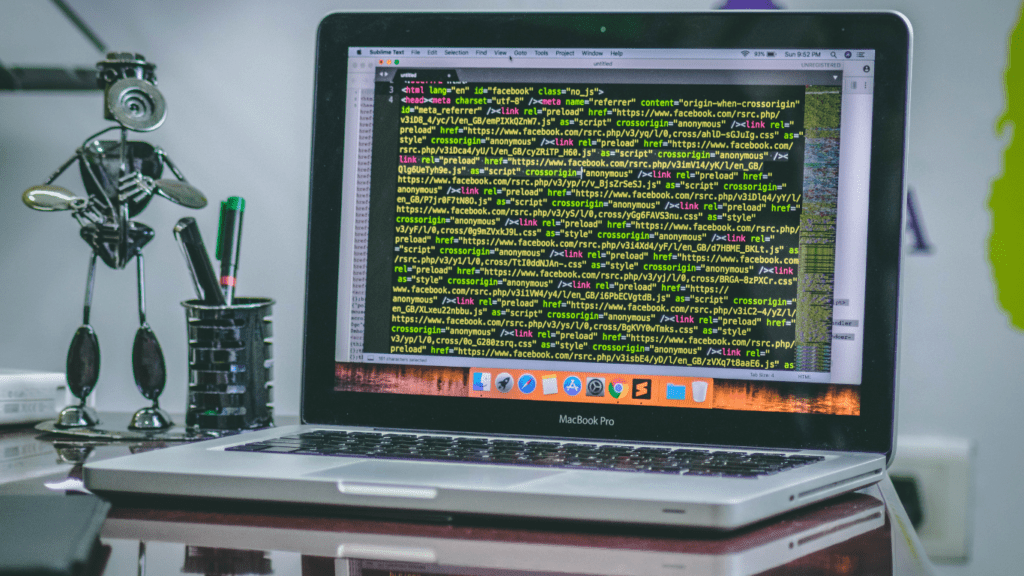
Segment Trees and Binary Indexed Trees
Segment Trees and Binary Indexed Trees (BIT) are crucial for efficiently handling range queries and updates.
Segment Trees provide a powerful method to perform range sum queries and range minimum queries.
They’re especially useful for static arrays where frequent updates occur. Segment Trees divide the array into segments, enabling O(log n) time complexity for both queries and updates.
Binary Indexed Trees, also known as Fenwick Trees, offer another way to handle prefix sum queries. They achieve O(log n) complexity for updates and queries.
BITs are simpler to implement than Segment Trees and are highly efficient for scenarios requiring cumulative frequency tables.
Advanced Graph Techniques
Advanced Graph Techniques streamline complex graph-related problems.
Tarjan’s Algorithm, which finds Strongly Connected Components (SCC), is indispensable for directed graphs. SCCs help identify cycles and components, making them crucial for solving problems like 2-SAT.
Another essential technique is the Floyd-Warshall algorithm. It calculates shortest paths between all pairs of nodes, suitable for dense graphs. Unlike Dijkstra’s algorithm, Floyd-Warshall handles negative edge weights effectively.
Lastly, Maximum Flow algorithms like the Ford-Fulkerson method and Edmonds-Karp algorithm solve network flow problems.
These methods are essential for applications involving maximum bipartite matching, circulation, and more complex network designs.
Competitive Programming Challenges and How to Tackle Them
Common Mistakes and How to Avoid Them
Many programmers struggle with competitive programming due to common pitfalls. Misunderstanding problem statements often leads to incorrect solutions.
- To avoid this, first, read problems thoroughly and highlight key points.
- Second, overlook edge cases, which is a frequent mistake. Always consider boundary conditions when developing your solution. Lastly, inefficient time complexity increases the risk of timing out.
- Practice analyzing algorithmic complexity to choose the most efficient solution available.
Practice Strategies and Time Management
Effective practice and time management are crucial for competitive programming. Consistent practice improves problem-solving speed.
Use platforms like:
- Codeforces
- LeetCode
- HackerRank
for daily challenges.
Analyze problems systematically by breaking them down into smaller, manageable subproblems. Focus on optimizing each component. Allocate specific time slots daily for practice to build a routine.
During competitions, manage time by dividing it among easy, medium, and hard problems. Start with easy ones to build confidence, then progress to more challenging tasks.
Maintain a balance between speed and accuracy to maximize performance.