Overview of RESTful APIs
What Are RESTful APIs?
RESTful APIs are a type of web service that follow the principles of Representational State Transfer (REST). REST relies on a stateless, client-server, cacheable communications protocol—most commonly, HTTP.
RESTful APIs enable the interaction between client and server by adhering to a uniform interface.
Resources, identified by URLs, can be manipulated through standard HTTP methods like GET, POST, PUT, and DELETE.
These principles ensure the efficient handling and transfer of data across the web.
Why Use RESTful APIs?
RESTful APIs have become a cornerstone in web development due to their simplicity and efficiency. They offer several advantages:
- Scalability: RESTful APIs handle multiple client requests and scale horizontally, which is essential for growing applications.
- Statelessness: Each API call from the client carries all necessary information, eliminating server session storage and making it easier to maintain.
- Flexibility: RESTful APIs can output various data formats such as JSON, XML, or plain text, making them versatile.
- Performance: The caching feature can improve API performance by reducing server load and lowering latency.
By following REST principles, developers ensure that APIs maintain consistency, ease of access, and improved performance, which significantly enhances the user experience.
Setting Up Your Development Environment
Necessary Tools and Software
For building RESTful APIs with Node.js and Express, some essential tools and software are required.
First, a code editor or Integrated Development Environment (IDE) is necessary. Popular options include Visual Studio Code, Sublime Text, and Atom.
Each offers features like syntax highlighting, debugging, and extensions to enhance development.
Version control tools are also important. Git is the most widely used version control system.
It facilitates code management and collaboration, with platforms like GitHub and GitLab for hosting repositories.
Postman is a useful tool for testing APIs. It allows you to send HTTP requests and inspect responses.
Additionally, using a package manager like npm (Node Package Manager) aids in managing project dependencies.
Installing Node.js and Express
To start, install Node.js from the official website.
Node.js is available for various operating systems, including Windows, macOS, and Linux. Download the installer and follow the installation instructions.
Once Node.js is installed, use npm to install Express globally. Open a terminal or command prompt and run the following command:
npm install -g express
Afterward, set up a new project directory. Navigate to the directory in your terminal and initialize a new Node.js project with:
npm init -y
Finally, install Express in the project directory by running:
npm install express
This concludes the basic setup. You’ve now prepared your development environment for building RESTful APIs with Node.js and Express.
Creating Your First RESTful API with Node.js
Initializing a Node.js Project
Setting up a Node.js project is essential for developing RESTful APIs. In your terminal, navigate to your chosen directory, then execute npm init -y
to generate a package.json
file.
This file manages project dependencies and metadata. Install Express next by running npm install express
.
mkdir my-restful-api
cd my-restful-api
npm init -y
npm install express
These commands create a directory, initialize a Node.js project, and install Express for building the API.
Building a Basic API with Express
To start building your API, create a file named index.js
in your project directory. This file contains the core logic and route handlers. Require Express and initialize an application instance.
const express = require('express');
const app = express();
const port = 3000;
app.get('/', (req, res) => {
res.send('Hello World!');
});
app.listen(port, () => {
console.log(`Server running at http://localhost:${port}`);
});
In this example, I created a simple GET route that responds with “Hello World!” when accessing the root URL. Start your server with node index.js
. Visit http://localhost:3000
in your browser to see the response.
Expand your API by adding more routes. For instance, create a file named routes.js
to handle CRUD operations.
const express = require('express');
const router = express.Router();
// In-memory data storage
let data = [];
router.get('/items', (req, res) => {
res.json(data);
});
router.post('/items', (req, res) => {
const item = req.body;
data.push(item);
res.status(201).json(item);
});
module.exports = router;
In index.js
, import routes.js
and mount it on the /api
path.
const express = require('express');
const app = express();
const routes = require('./routes');
const port = 3000;
app.use(express.json());
app.use('/api', routes);
app.listen(port, () => {
console.log(`Server running at http://localhost:${port}`);
});
Here, I set up a basic routing mechanism to manage items.
The GET and POST routes allow retrieving and adding items to an in-memory array. Use these routes to handle CRUD operations effectively.
Applying these steps creates a foundation for a RESTful API using Node.js and Express.
Advancing Your API
Implementing CRUD Operations
To advance my API, understanding CRUD operations is crucial.
CRUD stands for Create, Read, Update, and Delete, which are the core actions for interacting with a database. In Node.js with Express, I use route handlers to manage these operations.
- Create Operation: I use the
POST
HTTP method to add new data. For instance, I set up a route/api/items
and usereq.body
to access the data sent in the request. - Read Operation: Implementing the
GET
method allows me to retrieve data. For example, a route like/api/items/:id
fetches a specific item usingreq.params.id
. - Update Operation: I update existing data using the
PUT
method. On a route like/api/items/:id
, I grab the ID withreq.params.id
and update the data usingreq.body
. - Delete Operation: The
DELETE
method helps remove data. A route/api/items/:id
usesreq.params.id
to identify and delete the item.
Adding Middleware for Authentication
Authentication is essential for securing my API. Middleware in Express processes requests before they reach route handlers.
I use middleware to verify user credentials such:
- Install Middleware: First, I install packages like
jsonwebtoken
andbcryptjs
using npm. These help with token generation and password hashing. - Create Middleware Function: I create a function to check for tokens. The function looks like:
function authenticateToken(req, res, next) {
const authHeader = req.headers['authorization'];
const token = authHeader && authHeader.split(' ')[1];
if (!token) return res.sendStatus(401);
jwt.verify(token, process.env.ACCESS_TOKEN_SECRET, (err, user) => {
if (err) return res.sendStatus(403);
req.user = user;
next();
});
}
- Use Middleware in Routes: I apply this middleware to secure certain routes. For instance:
app.post('/api/items', authenticateToken, (req, res) => {
// route logic here
});
By implementing CRUD operations and middleware for authentication, I’ve effectively advanced my API, enhancing its functionality and security.
Best Practices in RESTful API Development
Error Handling Techniques
Effective error handling is essential in API development. It ensures users receive clear feedback when issues arise.
Utilize Express’s built-in error-handling middleware by defining a function with four arguments: err
, req
, res
, and next
.
When an error occurs, the middleware captures it and sends an appropriate response.
app.use((err, req, res, next) => {
console.error(err.stack);
res.status(500).json({ message: 'Internal Server Error' });
});
Define consistent error codes and messages for common issues. For instance, return a 404 status for not-found resources or a 400 status for bad user inputs. This consistency helps client applications handle errors more predictively.
Structuring API Endpoints
Organize API endpoints logically for simplicity and scalability.
Follow RESTful conventions by grouping routes according to resources. Use nouns for endpoints (e.g., /users
, /products
) to reflect resource-oriented architecture.
Apply intuitive HTTP methods:
- GET for retrieving resources,
- POST for creating new resources,
- PUT for updating existing resources,
- DELETE for removing resources.
Consider versioning your API endpoints (e.g., /api/v1/users
). This practice allows for backward-compatible changes, enabling clients to transition smoothly between API versions.
Define the routes clearly in Express:
const express = require('express');
const app = express();
app.get('/api/v1/users', (req, res) => {
res.json({ message: 'List of users' });
});
app.post('/api/v1/users', (req, res) => {
res.json({ message: 'User created' });
});
app.put('/api/v1/users/:id', (req, res) => {
res.json({ message: `User ${req.params.id} updated` });
});
app.delete('/api/v1/users/:id', (req, res) => {
res.json({ message: `User ${req.params.id} deleted` });
});
This clear, predictable structuring enhances the API’s usability and maintainability.
Testing RESTful APIs
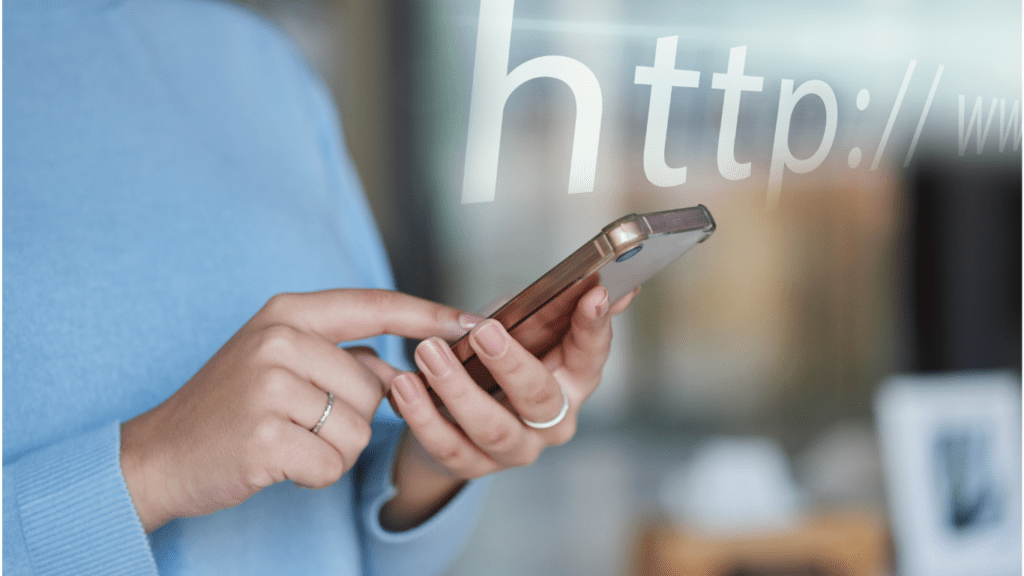
Effective testing ensures that RESTful APIs are functional, reliable, and secure. This section covers the tools and techniques for testing APIs built with Node.js and Express.
Tools for API Testing
Using specialized tools simplifies the API testing process. Postman, Jest, and Supertest are popular choices:
- Postman: Postman provides an intuitive interface for sending HTTP requests and viewing responses. It’s ideal for manual testing and automating test scripts through its extensive features.
- Jest: Jest, a JavaScript testing framework, integrates seamlessly with Node.js applications. It includes features like snapshot testing and a powerful mocking library.
- Supertest: Supertest simplifies HTTP assertions by providing a high-level abstraction for making HTTP requests. Combining it with Jest enables robust end-to-end testing.
Writing Test Cases
Writing effective test cases validates API endpoints under various conditions:
- Set Up Test Environment: Isolate testing environments from development to prevent data conflicts. Use tools like dotenv for managing environment variables in tests.
- Validate Status Codes: Confirm that each endpoint returns appropriate HTTP status codes for successful and erroneous requests. For instance, a
200 OK
for a successful read operation or a404 Not Found
for a missing resource. - Check Response Payloads: Ensure response bodies contain expected data structures and values. This includes verifying JSON keys and types match the API specification.
- Test Edge Cases: Cover edge cases by testing invalid or unexpected inputs. For example, inputting null values where integers are expected or sending malformed JSON requests.
Proper API testing guarantees the functionality and reliability of RESTful APIs built with Node.js and Express.
Deploying and Maintaining Your API
Deployment Options
Choosing the right deployment option for your RESTful API ensures optimal performance and availability.
When deploying an API built with Node.js and Express, several methods can be considered:
- Cloud Platforms: Services like AWS, Google Cloud, and Microsoft Azure offer robust features, including auto-scaling and load balancing. They enable seamless deployment via containerization with Docker or orchestration with Kubernetes.
- PaaS Solutions: Platforms such as Heroku and Vercel provide simplified deployment processes.
They often come with built-in tools for monitoring and scaling, making them ideal for smaller projects or rapid deployment needs. - On-Premises Servers: Deploying on on-premises servers might be suitable for organizations with specific compliance or security needs. This approach, though, typically requires more extensive infrastructure management and maintenance.
Monitoring and Scaling
Maintaining your API’s health and performance involves continuous monitoring and scaling. Effective monitoring can preemptively address potential issues, while scaling ensures your API can handle varying loads.
- Monitoring Tools: Tools like New Relic, DataDog, and Prometheus offer real-time performance insights. They help in tracking metrics like response times, error rates, and server utilization.
- Logging Services: Implementing services like Loggly and ELK Stack (Elasticsearch, Logstash, Kibana) ensures that API logs are stored, searched, and visualized effectively. Proper logging aids in diagnosing issues promptly.
- Auto-Scaling: Most cloud platforms provide auto-scaling features, allowing your API to adjust its resources based on the current load dynamically. This ensures high availability and performance even during peak traffic periods.
- Load Balancing: Ensures even distribution of incoming requests across multiple instances of your API, thus preventing any single instance from becoming a bottleneck. Cloud providers usually have built-in load balancing solutions.
Proactively deploying, monitoring, and scaling your Node.js and Express-based RESTful API ensures it remains reliable and performant under varying conditions.
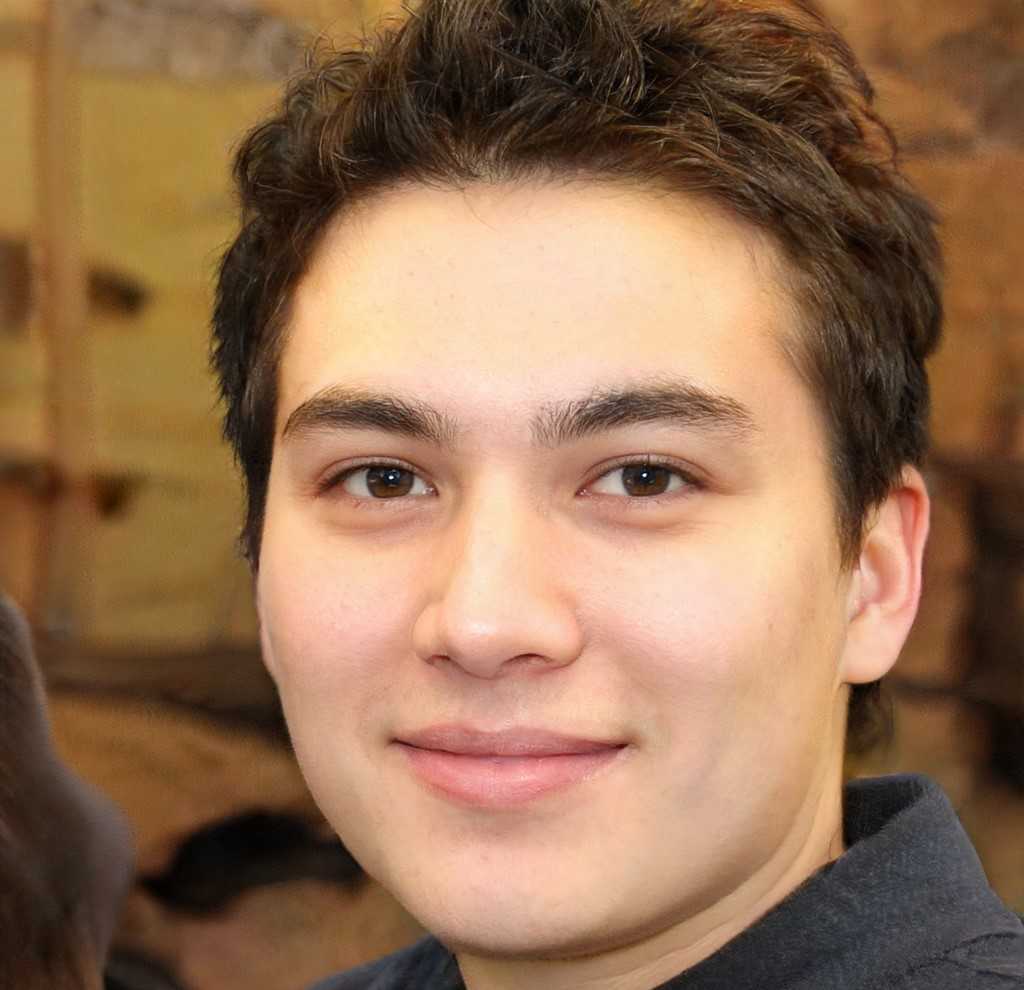
About the author:
Gerthann Stalcupy, the founder of your gtech colony , plays a pivotal role in shaping the direction and content of the platform. As the visionary behind the site. – Learn more